In this post I will be discussing the Scripts from the Mathematical and Number point of view. Although I have posted a more complex script (Simple Calculator) in the previous post, but on a user part it was difficult to understand and hence I thought to make you people learn the other useful side of learning in small packets.
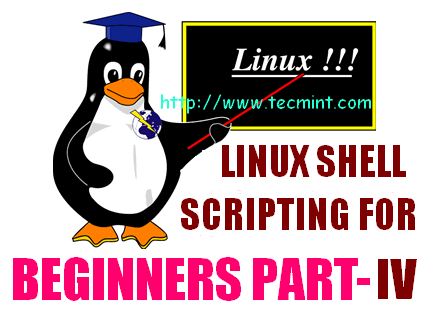
Prior to this article, three article of Shell Scripting Series are published and they are:
- Understand Linux Shell and Basic Shell Scripting – Part I
- 5 Shell Scripts to Learn Shell Programming – Part II
- Sailing Through The World of Linux BASH Scripting – Part III
Let’s start the further learning process with some new exciting scripts, start with Mathematics scripts:
Script 1: Additions
Create a file “Addition.sh” and chmod 755 to the script as described in previous post and run it.
#!/bin/bash echo “Enter the First Number: ” read a echo “Enter the Second Number: ” read b x=$(expr "$a" + "$b") echo $a + $b = $x
Sample Output
[root@tecmint ~]# vi Additions.sh [root@tecmint ~]# chmod 755 Additions.sh [root@tecmint ~]# ./Additions.sh “Enter the First Number: ” 12 “Enter the Second Number: ” 13 12 + 13 = 25
Script 2: Substraction
#!/bin/bash echo “Enter the First Number: ” read a echo “Enter the Second Number: ” read b x=$(($a - $b)) echo $a - $b = $x
Note: Here we replaced the expr and let the mathematical calculation be performed in shell.
Sample Output
[root@tecmint ~]# vi Substraction.sh [root@tecmint ~]# chmod 755 Substraction.sh [root@tecmint ~]# ./Substraction.sh “Enter the First Number: ” 13 “Enter the Second Number: ” 20 13 - 20 = -7
Script 3: Multiplication
So far you would be enjoying a lot, learning scripts in such an easy way, so the next in chronological order is Multiplication.
#!/bin/bash echo “Enter the First Number: ” read a echo “Enter the Second Number: ” read b echo "$a * $b = $(expr $a \* $b)"
Note: Yup! Here we didn’t put the value of multiplication in a variable but performed it directly in output statement.
Sample Output
[root@tecmint ~]# vi Multiplication.sh [root@tecmint ~]# chmod 755 Multiplication.sh [root@tecmint ~]# ./Multiplication.sh “Enter the First Number: ” 11 “Enter the Second Number: ” 11 11 * 11 = 121
Script 4: Division
Right! Next is Division, and again it is a very simple script. Check it Yourself.
#!/bin/bash echo “Enter the First Number: ” read a echo “Enter the Second Number: ” read b echo "$a / $b = $(expr $a / $b)"
Sample Output
[root@tecmint ~]# vi Division.sh [root@tecmint ~]# chmod 755 Division.sh [root@tecmint ~]# ./Division.sh “Enter the First Number: ” 12 “Enter the Second Number: ” 3 12 / 3 = 4
Script 5: Table
Fine! What after these basic mathematical operation. Lets write a script that prints table of any number.
#!/bin/bash echo “Enter The Number upto which you want to Print Table: ” read n i=1 while [ $i -ne 10 ] do i=$(expr $i + 1) table=$(expr $i \* $n) echo $table done
Sample Output
[root@tecmint ~]# vi Table.sh [root@tecmint ~]# chmod 755 Table.sh [root@tecmint ~]# ./Table.sh “Enter The Number upto which you want to Print Table: ” 29 58 87 116 145 174 203 232 261 290
Script 6: EvenOdd
We as a child always have carried out calculation to find if the number is odd or even. Won’t it be a good idea to implement it in script.
#!/bin/bash echo "Enter The Number" read n num=$(expr $n % 2) if [ $num -eq 0 ] then echo "is a Even Number" else echo "is a Odd Number" fi
Sample Output
[root@tecmint ~]# vi EvenOdd.sh [root@tecmint ~]# chmod 755 EvenOdd.sh [root@tecmint ~]# ./EvenOdd.sh Enter The Number 12 is a Even Number
[root@tecmint ~]# ./EvenOdd.sh Enter The Number 11 is a Odd Number
Script 7: Factorial
Next is to find the Factorial.
#!/bin/bash echo "Enter The Number" read a fact=1 while [ $a -ne 0 ] do fact=$(expr $fact \* $a) a=$(expr $a - 1) done echo $fact
Sample Output
[root@tecmint ~]# vi Factorial.sh [root@tecmint ~]# chmod 755 Factorial.sh [root@tecmint ~]# ./Factorial.sh Enter The Number 12 479001600
You may now relax with a feeling that calculating 12*11*10*9*7*7*6*5*4*3*2*1 would be more difficult than a simple script as produced above. Think of the situation where you require to find 99! or something like that. Sure! This script will be very much handy in that situation.
Script 8: Armstrong
Armstrong Number! Ohhh You forget what an Armstrong Number is. Well an Armstrong number of three digits is an integer such that the sum of the cubes of its digits is equal to the number itself. For example, 371 is an Armstrong number since 3**3 + 7**3 + 1**3 = 371.
#!/bin/bash echo "Enter A Number" read n arm=0 temp=$n while [ $n -ne 0 ] do r=$(expr $n % 10) arm=$(expr $arm + $r \* $r \* $r) n=$(expr $n / 10) done echo $arm if [ $arm -eq $temp ] then echo "Armstrong" else echo "Not Armstrong" fi
Sample Output
[root@tecmint ~]# vi Armstrong.sh [root@tecmint ~]# chmod 755 Armstrong.sh [root@tecmint ~]# ./Armstrong.sh Enter A Number 371 371 Armstrong
[root@tecmint ~]# ./Armstrong.sh Enter A Number 123 36 Not Armstrong
Script 9: Prime
The last script is to distinguish whether a number is prime or not.
#!/bin/bash echo “Enter Any Number” read n i=1 c=1 while [ $i -le $n ] do i=$(expr $i + 1) r=$(expr $n % $i) if [ $r -eq 0 ] then c=$(expr $c + 1) fi done if [ $c -eq 2 ] then echo “Prime” else echo “Not Prime” fi
Sample Output
[root@tecmint ~]# vi Prime.sh [root@tecmint ~]# chmod 755 Prime.sh [root@tecmint ~]# ./Prime.sh “Enter Any Number” 12 “Not Prime”
That’s all for now. In our very next article we will be covering other mathematical programs in the shell Scripting programming language. Don’t forget to mention your views regarding article in the Comment section. Like and share us and help us spread. Come Visiting tecmint.com for News and articles relating to FOSS. Till then Stay tuned.
thnks